本文深入分析下Executor一些核心原理
SqlSession对数据库的操作都是委托给Executor.下面我们剖析下Executor的核心原理。
前述
Executor 上承SqlSession 下接StatementHandler。处于Mybatis体系的核心,深入理解Executor的实现原理,对实际开发会更有帮助。
Executor接口
1 | public interface Executor { |
类关系图
实际只有五种:
- SimpleExecutor
- ReuseExecutor
- ClosedExecutor
- CachingExecutor
- BatchExecutor
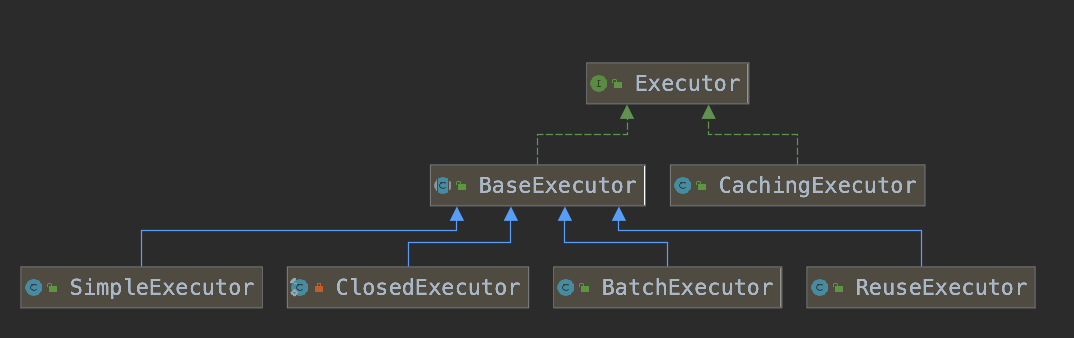
注意:以上执行器的生命周期都是在SqlSession生命周期内
SimpleExecutor
执行update/select 每次都创建一个StatementHandler,使用完就关闭。
CloseExecutor
私有类,没有什么实质实现.
ReuseExecutor
执行update/select 创建了StatementHandler之后,使用完,不关闭,缓存起来。
BatchExecutor
执行update. 不支持批量select. 所有的sql都会调用StatementHandler.addBatch(),等待executeBatch().
这里特别要注意下: 每个statement都可能是一批sql. BatchExecutor是维护了多个statement。借用大佬的解释BatchExecutor 维护了多个桶,每个桶里面有多个自己的SQL,就像苹果蓝里装了很多苹果,番茄蓝里装了很多番茄,最后,再统一倒进仓库(统一执行)。
CacheExecutor
基于装饰器模式,如果有缓存就直接返回缓存,如果没有缓存就委托给SimpleExecutor
|ReuseExecutor
|BatchExecutor
.这三种执行器执行。
部分源码分析
BaseExecutor 抽象类
部分代码
1 | @Override |
典型的模板模式,具体的实现交给下面的子类来实现.
SimpleExecutor
很简单,就是创建Statement,然后执行完后,就关闭掉。 其他方法可以看下具体实现,原理都一样.
1 | @Override |
注意:SimpleExecutor不支持doFlushStatements(批量刷新statement),所以是直接返回的一个空列表.
1 | @Override |
ReuseExecutor
1 | @Override |
注意: 因为ReuseExecutor 缓存了StatementHandler,所以doFlushStatements, 会关闭之前缓存的StatementHandler,然后返回一个空列表
1 | @Override |
BatchExecutor
1 | // 保存需要执行的statementList, 为之后批次执行做准备 |
需要注意的是: 如果SQL是AABBAA->那么就会有三个statement.
调用时序图
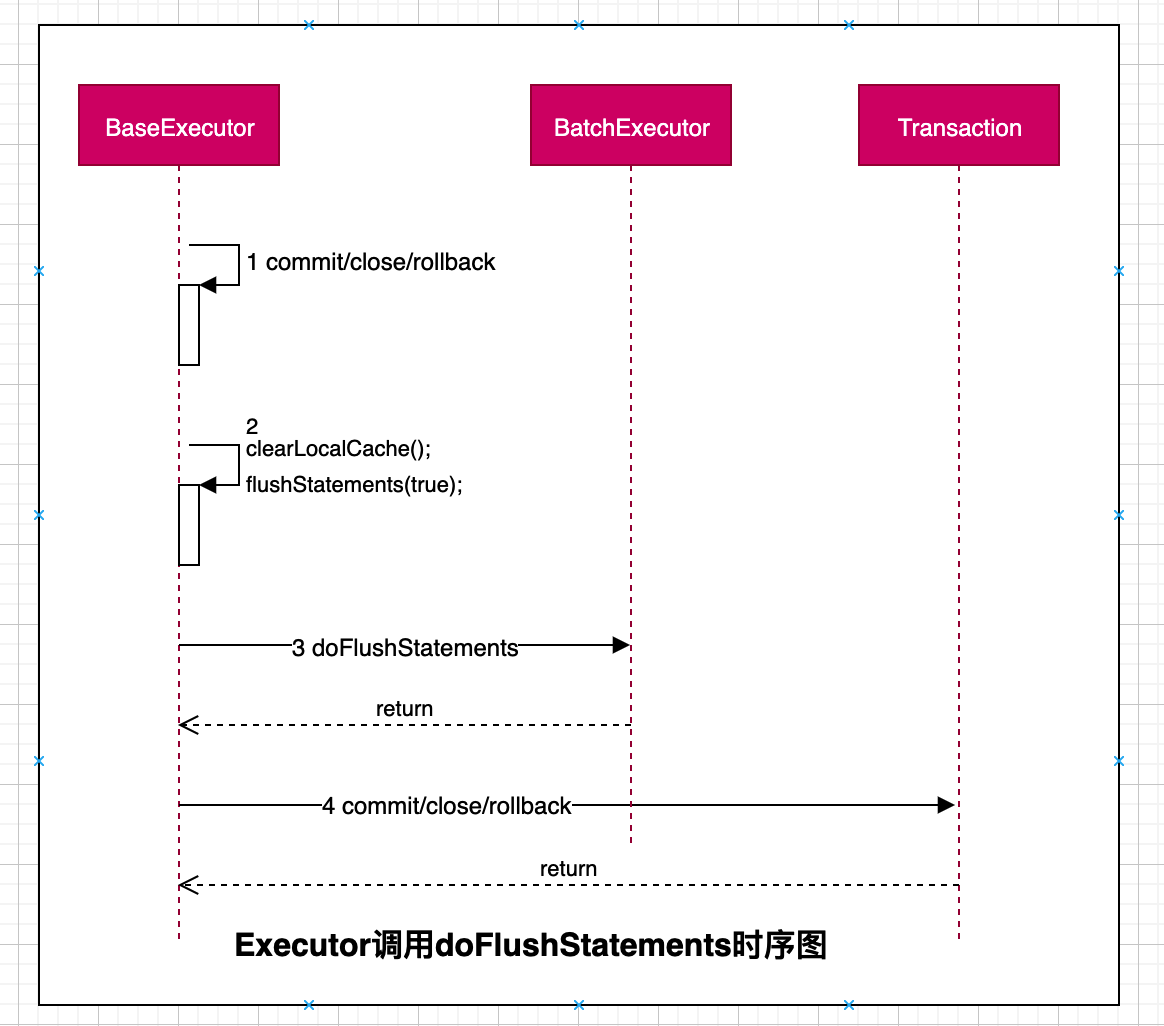
CachingExecutor
装饰模式,实际是使用的SimpleExecutor, ReusedExecutor, BatchExecutor 三种之一。
tips
- Executor什么时候生成
- 在创建SqlSession的时候,随之生成。
- Executor 默认方式是什么? 可否指定
- 默认是SimpleExecutor
- 可以指定,有下面两种方式
- 通过SqlSessionFactory.openSession() 传入ExecutorType
- 通过xml
来指定